C# : Multicast delegates : Part 15
- A delegate pointing to more than one function is called Multicast Delegate.
- When this delegate is invoked all the functions pointing to are invoked.
- If the delegate has return type other than void and if the delgate is multicast delegate than only the value of last invoked method will be returned.
- Multicast delegate makes observer design pattern very simple.
- Multicast delegate can be created by two ways
- =
- +=
- In multicast delegate all the method assigned to delegate should have same signature (return type).
Example
namespace DelegatesMulticast
{
class Program
{
public delegate void PrintDelegate();
static void Main(string[] args)
{
//Approach 1
Console.WriteLine("Approach 1 '='");
PrintDelegate del1, del2, del3, del4;
del1 = new PrintDelegate(Print1);
del2 = new PrintDelegate(Print2);
del3 = new PrintDelegate(Print3);
del4 = del1 + del2 + del3;
del4();
//Approach 2
Console.WriteLine("Approach 2 '+='");
PrintDelegate del = new PrintDelegate(Print1);
del += Print2;
del += Print3;
del();
}
public static void Print1()
{
Console.WriteLine("Invoked from Method 1");
}
public static void Print2()
{
Console.WriteLine("Invoked from Method 2");
}
public static void Print3()
{
Console.WriteLine("Invoked from Method 3");
}
}
}
Output
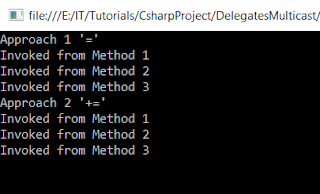
No comments