C# : Delegates : Part 14
- A delegate is a type safe function pointer ( It points towards a function with same signature)
- It is called function pointer because when you invoke a delegate the function will be invoked.
- It is called type safe because the signature of method should be same to the signature of delegate declared, and if the signature does not matches it gives compile time error.
- Initialization of delegate is very simple, similar to class. Create the instance of the delegate and pass the name of function as parameter to which you want to point the delgate.
namespace Delegate
{
public delegate void HelloWorldFunctionPointer(string message);
class Program
{
static void Main(string[] args)
{
HelloWorldFunctionPointer del = new HelloWorldFunctionPointer(HelloWorld);
del("How are you.");
}
public static void HelloWorld(string message)
{
Console.WriteLine(message);
}
}
}
Why use delegates ?
- It provides flexibility & reuseability in programming.
namespace DelegateFlexibilityReuseability
{
public delegate bool PrintTopperDelagate(Student stu);
class Program
{
static void Main(string[] args)
{
List<Student> ListStudent = new List<Student>();
ListStudent.Add(new Student { Name = "Amol", Class = "Eigth", RollNum = 4, Percentage = 93 });
ListStudent.Add(new Student { Name = "Ritesh", Class = "Eigth", RollNum = 6, Percentage = 67 });
ListStudent.Add(new Student { Name = "Pandurang", Class = "Eigth", RollNum = 4, Percentage = 90 });
ListStudent.Add(new Student { Name = "Hansraj", Class = "Eigth", RollNum = 4, Percentage = 89 });
ListStudent.Add(new Student { Name = "Dinesh", Class = "Eigth", RollNum = 4, Percentage = 98 });
PrintTopperDelagate del = new PrintTopperDelagate(FindTopper);
Student.PrintTopper(ListStudent, del);
}
public static bool FindTopper(Student objStudent)
{
if (objStudent.Percentage > 90)
return true;
else
return false;
}
}
public class Student
{
public string Name { get; set; }
public string Class { get; set; }
public int RollNum { get; set; }
public int Percentage { get; set; }
public static void PrintTopper(List<Student> ListStu, PrintTopperDelagate IsTopper)
{
foreach (var item in ListStu)
{
if (IsTopper(item))
Console.WriteLine(item.Name);
}
}
}
}
Output
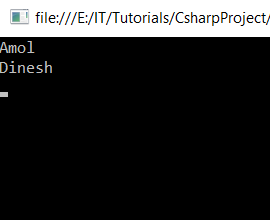
No comments